The Most Beautiful Piece of Code That Prints 42
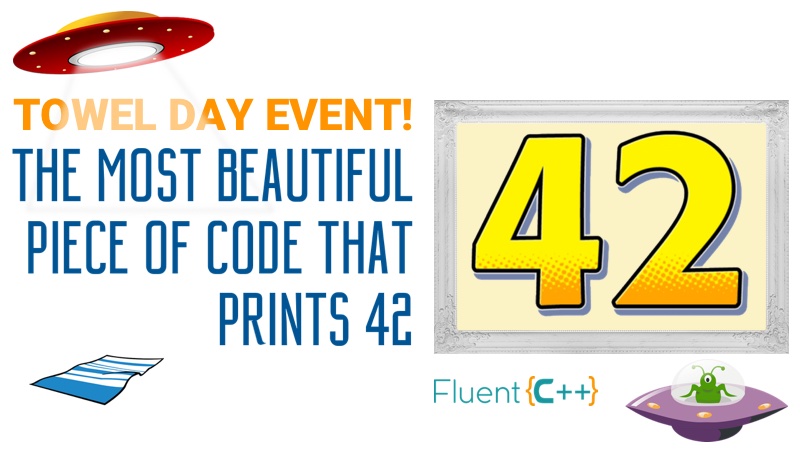
Two weeks from now, on the 25th of May, is a very important event in the geek culture: Towel Day, and I’d love to celebrate it with you on Fluent C++! EDIT: check out the most beautiful piece of code that print 42 here! Towel day? Towel day is a yearly tribute to the fiction […]